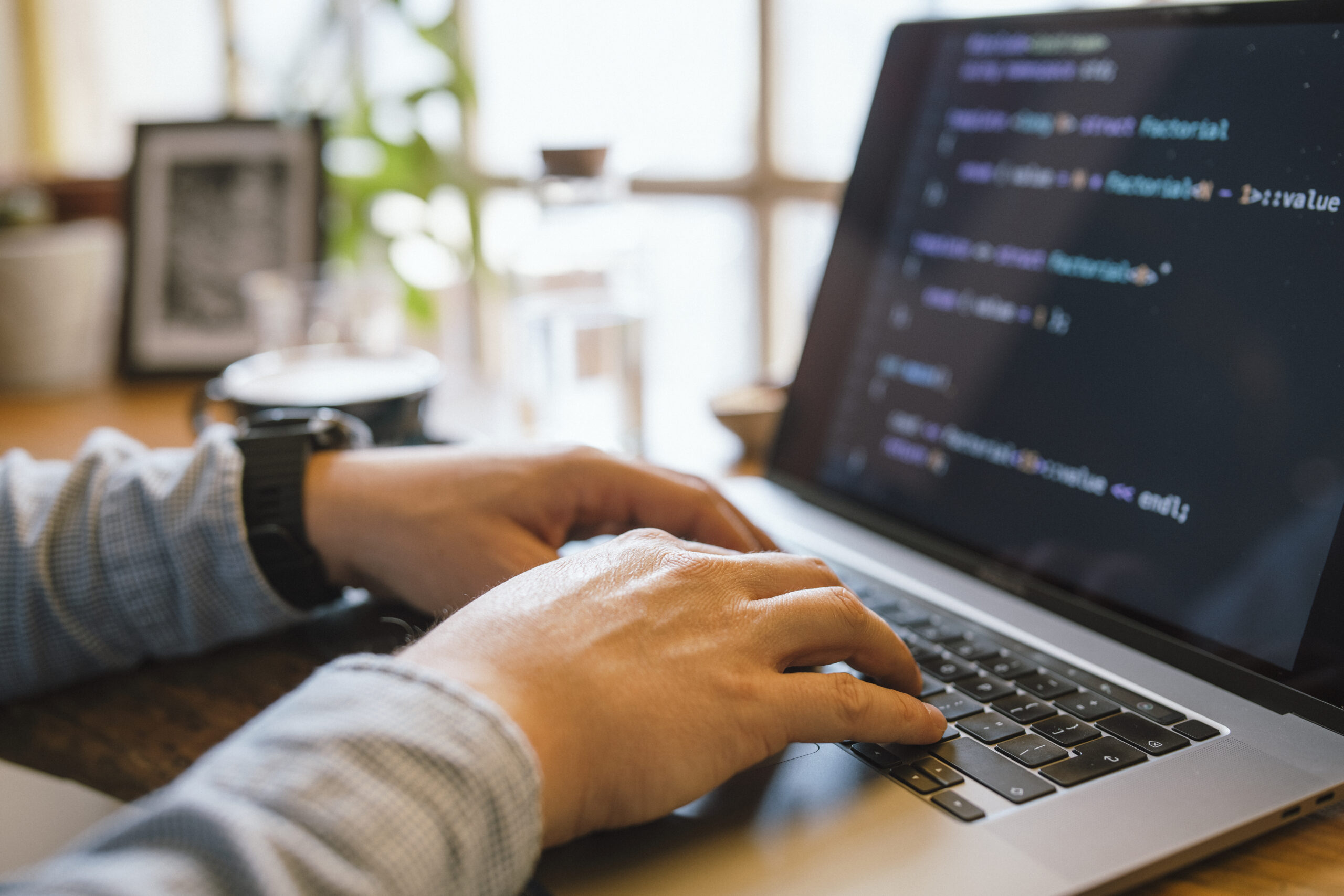
Debugging is Probably the most necessary — yet frequently neglected — competencies in a developer’s toolkit. It's not just about fixing damaged code; it’s about understanding how and why issues go Incorrect, and Understanding to Consider methodically to resolve troubles successfully. Whether you are a starter or simply a seasoned developer, sharpening your debugging skills can save several hours of irritation and dramatically improve your efficiency. Here i will discuss quite a few procedures that will help builders degree up their debugging recreation by me, Gustavo Woltmann.
Master Your Resources
One of many quickest means builders can elevate their debugging capabilities is by mastering the equipment they use each day. While crafting code is just one Section of advancement, understanding how to connect with it properly in the course of execution is equally significant. Present day improvement environments occur Outfitted with potent debugging abilities — but a lot of developers only scratch the floor of what these equipment can do.
Acquire, by way of example, an Integrated Enhancement Setting (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These instruments permit you to established breakpoints, inspect the value of variables at runtime, move by way of code line by line, as well as modify code to the fly. When employed the right way, they Permit you to observe accurately how your code behaves for the duration of execution, which is priceless for monitoring down elusive bugs.
Browser developer equipment, which include Chrome DevTools, are indispensable for front-close developers. They assist you to inspect the DOM, check community requests, see authentic-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and network tabs can convert irritating UI troubles into workable tasks.
For backend or technique-amount developers, equipment like GDB (GNU Debugger), Valgrind, or LLDB give deep Manage in excess of running processes and memory management. Mastering these tools might have a steeper Mastering curve but pays off when debugging performance concerns, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Command systems like Git to comprehend code historical past, come across the precise instant bugs were introduced, and isolate problematic adjustments.
Eventually, mastering your instruments usually means likely beyond default settings and shortcuts — it’s about creating an intimate understanding of your advancement setting making sure that when challenges crop up, you’re not shed at the hours of darkness. The better you realize your resources, the more time you are able to shell out fixing the actual difficulty as an alternative to fumbling by way of the method.
Reproduce the trouble
The most vital — and sometimes ignored — actions in effective debugging is reproducing the problem. Before leaping in the code or generating guesses, developers need to produce a reliable setting or situation exactly where the bug reliably seems. Without reproducibility, correcting a bug gets a recreation of opportunity, often bringing about wasted time and fragile code changes.
The initial step in reproducing a difficulty is gathering just as much context as you can. Inquire questions like: What steps resulted in The difficulty? Which setting was it in — improvement, staging, or output? Are there any logs, screenshots, or error messages? The greater depth you've, the a lot easier it gets to isolate the exact ailments below which the bug takes place.
As soon as you’ve collected plenty of info, seek to recreate the trouble in your neighborhood setting. This may indicate inputting the same knowledge, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, think about producing automated exams that replicate the sting situations or point out transitions concerned. These assessments not only support expose the problem but in addition reduce regressions in the future.
Often, the issue could be ecosystem-particular — it would transpire only on certain working devices, browsers, or less than specific configurations. Employing applications like Digital machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating these types of bugs.
Reproducing the issue isn’t only a phase — it’s a way of thinking. It requires patience, observation, as well as a methodical technique. But when you finally can continuously recreate the bug, you're currently halfway to repairing it. By using a reproducible circumstance, You may use your debugging tools much more successfully, check prospective fixes securely, and talk a lot more Obviously using your crew or end users. It turns an abstract grievance into a concrete challenge — Which’s where builders prosper.
Examine and Fully grasp the Mistake Messages
Error messages are frequently the most precious clues a developer has when one thing goes Incorrect. Instead of seeing them as frustrating interruptions, builders really should understand to treat mistake messages as immediate communications from your method. They often let you know precisely what happened, where by it took place, and at times even why it happened — if you know the way to interpret them.
Start out by reading through the message diligently As well as in complete. Many builders, particularly when under time force, glance at the first line and promptly commence making assumptions. But further inside the mistake stack or logs may possibly lie the accurate root induce. Don’t just duplicate and paste error messages into search engines — examine and comprehend them to start with.
Split the mistake down into elements. Can it be a syntax error, a runtime exception, or simply a logic error? Will it level to a selected file and line amount? What module or functionality induced it? These thoughts can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re working with. Mistake messages in languages like Python, JavaScript, or Java usually abide by predictable patterns, and Mastering to recognize these can dramatically increase your debugging method.
Some glitches are vague or generic, and in All those cases, it’s vital to look at the context in which the error transpired. Look at associated log entries, input values, and up to date variations within the codebase.
Don’t forget about compiler or linter warnings possibly. These often precede greater difficulties and supply hints about potential bugs.
In the end, error messages are not your enemies—they’re your guides. Understanding to interpret them effectively turns chaos into clarity, encouraging you pinpoint problems more quickly, lessen debugging time, and turn into a extra economical and confident developer.
Use Logging Wisely
Logging is The most highly effective applications in a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, assisting you realize what’s taking place beneath the hood with no need to pause execution or stage with the code line by line.
An excellent logging method begins with realizing what to log and at what degree. Typical logging ranges consist of DEBUG, Information, WARN, Mistake, and Lethal. Use DEBUG for specific diagnostic data for the duration of growth, Data for basic occasions (like effective start-ups), Alert for probable difficulties that don’t split the application, Mistake for genuine troubles, and FATAL when the procedure can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure significant messages and slow down your system. Deal with essential activities, state improvements, input/output values, and important final decision points in the code.
Structure your log messages clearly and continually. Contain context, such as timestamps, ask for IDs, and function names, so it’s simpler to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
During debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without halting the program. They’re Primarily worthwhile in creation environments where by stepping by way of code isn’t possible.
Moreover, use logging frameworks and tools (like Log4j, Winston, or Python’s logging module) that assist log rotation, filtering, and integration with checking dashboards.
In the end, clever logging is about balance and clarity. By using a perfectly-believed-out logging tactic, you are able to decrease the time it's going to take to spot concerns, attain deeper visibility into your programs, and Enhance the In general maintainability and reliability of the code.
Assume Like a Detective
Debugging is not only a complex undertaking—it is a type of investigation. To proficiently identify and repair bugs, developers ought to solution the process like a detective fixing a thriller. This way of thinking allows break down complicated concerns into workable sections and abide by clues logically to uncover the foundation cause.
Begin by collecting evidence. Consider the indicators of the situation: mistake messages, incorrect output, or general performance challenges. Just like a detective surveys a crime scene, collect as much relevant info as you are able to without having jumping to conclusions. Use logs, check instances, and user reports to piece together a clear picture of what’s happening.
Next, variety hypotheses. Talk to you: What can be producing this habits? Have any alterations just lately been created for the codebase? Has this problem occurred right before underneath related conditions? The aim would be to slender down options and discover prospective culprits.
Then, check your theories systematically. Try to recreate the situation in the controlled environment. When you suspect a selected operate or component, isolate it and validate if The problem persists. Similar to a detective conducting interviews, question your code queries and Enable the final results lead you nearer to the truth.
Pay back near interest to tiny aspects. Bugs typically hide from the minimum envisioned spots—like a missing semicolon, an off-by-one mistake, or perhaps a race ailment. Be here comprehensive and patient, resisting the urge to patch The problem without entirely comprehending it. Momentary fixes might cover the real dilemma, just for it to resurface later on.
Last of all, preserve notes on Anything you experimented with and acquired. Just as detectives log their investigations, documenting your debugging course of action can save time for foreseeable future issues and aid Some others comprehend your reasoning.
By contemplating similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be more practical at uncovering concealed issues in sophisticated devices.
Write Tests
Creating assessments is among the simplest ways to enhance your debugging capabilities and Over-all enhancement efficiency. Tests not just aid catch bugs early and also function a security Web that offers you self-confidence when producing alterations on your codebase. A very well-analyzed software is much easier to debug as it helps you to pinpoint exactly where and when a problem occurs.
Start with device checks, which focus on individual capabilities or modules. These small, isolated checks can immediately expose irrespective of whether a specific piece of logic is working as expected. When a exam fails, you straight away know where by to glance, appreciably reducing some time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear just after Earlier getting set.
Next, combine integration exams and finish-to-close assessments into your workflow. These aid ensure that many portions of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complex devices with several factors or companies interacting. If some thing breaks, your checks can let you know which Element of the pipeline failed and less than what problems.
Writing assessments also forces you to Imagine critically about your code. To check a function adequately, you'll need to be familiar with its inputs, anticipated outputs, and edge conditions. This degree of being familiar with By natural means potential customers to better code framework and much less bugs.
When debugging a problem, producing a failing test that reproduces the bug might be a robust first step. After the take a look at fails regularly, it is possible to focus on fixing the bug and look at your exam pass when The problem is solved. This approach ensures that precisely the same bug doesn’t return Down the road.
In short, composing assessments turns debugging from the frustrating guessing sport into a structured and predictable course of action—encouraging you catch much more bugs, more rapidly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to become immersed in the situation—gazing your screen for hours, striving Option just after solution. But Probably the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new viewpoint.
When you're as well close to the code for as well lengthy, cognitive fatigue sets in. You may start overlooking obvious errors or misreading code that you wrote just several hours before. With this condition, your brain gets to be much less efficient at trouble-resolving. A short walk, a espresso crack, or maybe switching to a distinct activity for 10–15 minutes can refresh your focus. Lots of builders report locating the root of a dilemma once they've taken time for you to disconnect, letting their subconscious do the job from the track record.
Breaks also help reduce burnout, In particular in the course of lengthier debugging classes. Sitting down in front of a monitor, mentally caught, is not only unproductive and also draining. Stepping away helps you to return with renewed Electricity as well as a clearer mindset. You would possibly abruptly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you in advance of.
In the event you’re trapped, an excellent general guideline is always to established a timer—debug actively for 45–sixty minutes, then take a 5–ten minute split. Use that point to move all-around, stretch, or do a thing unrelated to code. It may sense counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your point of view, and helps you avoid the tunnel eyesight That always blocks your development. Debugging is really a mental puzzle, and relaxation is an element of solving it.
Find out From Each individual Bug
Each bug you come across is a lot more than simply a temporary setback—It is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile when you go to the trouble to reflect and analyze what went Incorrect.
Commence by asking by yourself some vital thoughts as soon as the bug is fixed: What caused it? Why did it go unnoticed? Could it happen to be caught earlier with much better methods like unit testing, code reviews, or logging? The responses normally expose blind places as part of your workflow or knowledge and make it easier to Make more robust coding practices relocating forward.
Documenting bugs may also be a great habit. Keep a developer journal or maintain a log in which you Observe down bugs you’ve encountered, how you solved them, and Everything you discovered. Over time, you’ll begin to see designs—recurring concerns or typical mistakes—you could proactively stay clear of.
In staff environments, sharing Whatever you've discovered from the bug with the peers can be In particular strong. Regardless of whether it’s through a Slack information, a short create-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts crew efficiency and cultivates a more robust Studying society.
Far more importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll get started appreciating them as vital parts of your progress journey. In the end, many of the greatest builders aren't those who write best code, but those that repeatedly learn from their problems.
Eventually, Each and every bug you take care of adds a whole new layer to your ability established. So future time you squash a bug, take a minute to replicate—you’ll come away a smarter, additional able developer due to it.
Summary
Improving upon your debugging abilities normally takes time, observe, and patience — nevertheless the payoff is big. It makes you a more successful, self-assured, and capable developer. The following time you happen to be knee-deep inside of a mysterious bug, remember: debugging isn’t a chore — it’s an opportunity to become greater at Anything you do.